Moq
When properly designed, your project will consist of a number of classes, where each adheres to the Single Responsibility Principle. There will be a good amount of collaboration between your classes, and each will depend on the other doing its job. Here is a simple, contrived example that should both explain the problem, and how "mocking" and Moq solve the problem.Let's start out with an interface for a fancy Weather Service:
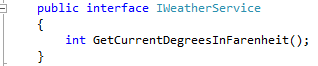
Why an interface? Well, you are "programming to an interface rather than an implementation" aren't you? If not, learn that part FIRST and come back to this when you're done... At any rate, we can now take a look at one very complex implementation of that interface:
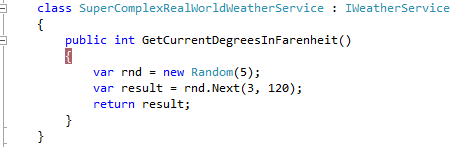
That could easily be some third party component or web service or such. Something we PAY for since it's so complex and awesome. Meanwhile, back in OUR code, we want to use the output of that service inside some business class of our own. Like so:
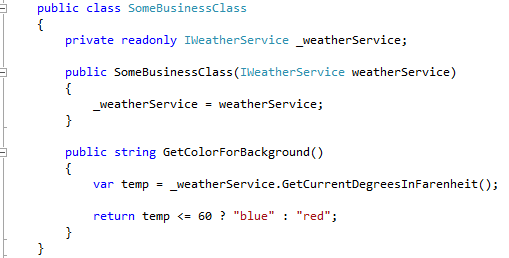
And, of course, we want to TEST our logic, right? The first problem in testing has been solved already - the business class that we want to test already adheres to the Single Responsibility Principle. It is using a practice called "Dependency Injection" so that it depends on an implementation of IWeatherService - but it does not know or care where that instance comes from. That is someone else's responsibility. Good stuff. Again, if this part isn't crystal clear to you - LEARN THAT FIRST, then come back to this.
The second problem in testing is staring at us right in the face, however. Consistency. Predictability. Tests must be predictable - with consistent results. If we were to do this:
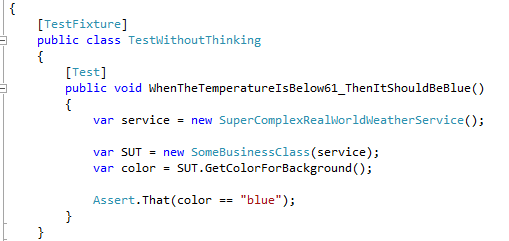
Then, how would we test? All summer long the above code would fail, but it would work great in the winter! The opposite would be true in the summer. We can't use the "real" Weather Service to supply data for our test - it is unreliable. It will give us a different result every time - or as often as the temperature changes. Unacceptable.
Enter the concept of "mocking." Sometimes called "fakes" or "test stubs." OMG please don't get into a religious war worrying about if a particular case calls for a mock versus a stub or even a "test double." Just get the concept, and move on... That concept being, that "in order to provide consistent inputs for our subject under test, we need to create an instance of the dependency that behaves the way we tell it to."
In code, this looks something like:
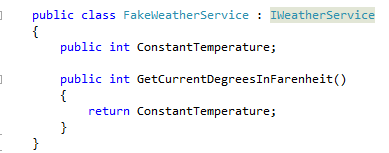
So, we've created another class, that implements the same interface as the real weather service. Notice that even though it implements the interface, it also has another field - the ConstantTemperature. As you can see from looking at it, this class simply returns the value you have told it to return. No hitting a real thermometer or web service or anything. How will we use that?
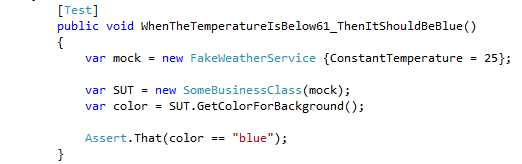
So, we created an instance of it, set the desired temperature, and tested that "our code" behaved correctly for the given input. Nice, neat, and simple. But not simple enough. We had to create a whole other class just for the purpose of this test. While that works, it leaves something to be desired. This is where mocking tools like Moq come in.... Here is the same test, using Moq:
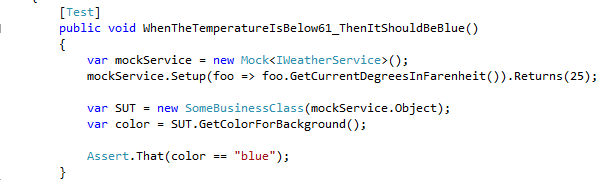
There is a little bit of crazy syntax to learn, but.... you didn't have to create another class! In practice, there are a TON of ways to use this, and once you learn the syntax you'll never want to go back. The documentation is pretty good too.
Enjoy!